Breaking Down Python's Built in Function `zip`
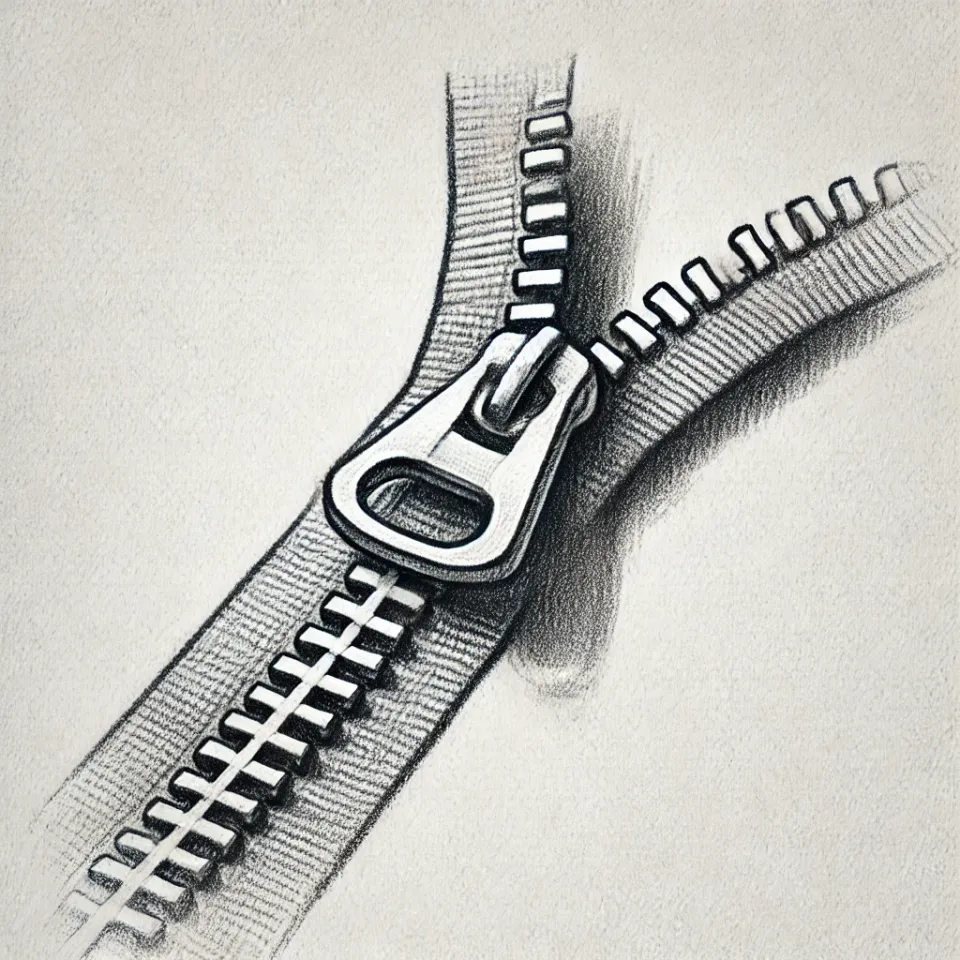
ZIP
zip()
is more efficient in both memory usage and simplicity, and it's preferred for combining objects in Python, especially when working with multiple iterables.
Key points about zip()
:
- It combines multiple iterables (such as lists, tuples, or strings) element by element.
- It returns an iterator of tuples, where each tuple contains one element from each of the input iterables.
- The function stops when the shortest iterable is exhausted, so if the input lists are of different lengths, the result will be truncated to match the shortest list.
Example:
a = [1, 2, 3]
b = ['a', 'b', 'c']
zipped = zip(a, b)
print(list(zipped))
Output:
[(1, 'a'), (2, 'b'), (3, 'c')]
In the case below, zip()
is used to combine elements from the names
and primary_types
lists.
names = ['User1', 'User2', 'User3', 'User4', 'User5']
primary_types = ['Electric', 'Fire', 'Flood']
# Combine the first five items from names and the first three from primary_types
differing_lengths = [*zip(names[:5], primary_types[:3])]
# Print the result
print(*differing_lengths, sep='\n')
Step-by-step Breakdown
- List Declaration
names = ['User1', 'User2', 'User3', 'User4', 'User5']
primary_types = ['Electric', 'Fire', 'Flood']
- Two lists are declared:
names
contains five user names:['User1', 'User2', 'User3', 'User4', 'User5']
.primary_types
contains three types:['Electric', 'Fire', 'Flood']
.
- Slicing Lists:
names[:5] # ['User1', 'User2', 'User3', 'User4', 'User5']
primary_types[:3] # ['Electric', 'Fire', 'Flood']
names[:5]
retrieves the first five elements of thenames
list (in this case, all elements).primary_types[:3]
retrieves the first three elements of theprimary_types
list.
- Using
zip
:
zip(names[:5], primary_types[:3])
- The
zip()
function pairs up elements from both lists based on the shortest list's length. - Since
primary_types
has only 3 elements, only the first 3 items fromnames
are zipped:('User1', 'Electric')
('User2', 'Fire')
('User3', 'Flood')
- The resulting pairs are returned as tuples in a
zip
object.
- Unpacking and Creating a List:
differing_lengths = [*zip(names[:5], primary_types[:3])]
- The
[*zip(...)]
syntax unpacks thezip
object and turns it into a list of tuples. - The result will be:
[('User1', 'Electric'), ('User2', 'Fire'), ('User3', 'Flood')]
- Printing the Result:
python print(*differing_lengths, sep='\n')
print(*differing_lengths)
unpacks the list, printing each tuple separately.sep='\n'
ensures that each tuple is printed on a new line.- The output will be
('User1', 'Electric')
('User2', 'Fire')
('User3', 'Flood')
Summary
This code takes two lists (names
and primary_types
), combines the first five names and the first three primary types using zip()
, and prints each pair of items from the two lists line by line.